go-events alternatives and similar packages
Based on the "Messaging" category.
Alternatively, view go-events alternatives based on common mentions on social networks and blogs.
-
sarama
DISCONTINUED. Sarama is a Go library for Apache Kafka. [Moved to: https://github.com/IBM/sarama] -
Centrifugo
Scalable real-time messaging server in a language-agnostic way. Self-hosted alternative to Pubnub, Pusher, Ably. Set up once and forever. -
APNs2
⚡ HTTP/2 Apple Push Notification Service (APNs) push provider for Go — Send push notifications to iOS, tvOS, Safari and OSX apps, using the APNs HTTP/2 protocol. -
Uniqush-Push
Uniqush is a free and open source software system which provides a unified push service for server side notification to apps on mobile devices. -
amqp
An AMQP 0-9-1 Go client maintained by the RabbitMQ team. Originally by @streadway: `streadway/amqp` -
Chanify
Chanify is a safe and simple notification tools. This repository is command line tools for Chanify. -
PingMe
PingMe is a CLI which provides the ability to send messages or alerts to multiple messaging platforms & email. -
emitter
Emits events in Go way, with wildcard, predicates, cancellation possibilities and many other good wins -
Bus
🔊Minimalist message bus implementation for internal communication with zero-allocation magic on Emit -
go-mq
Declare AMQP entities like queues, producers, and consumers in a declarative way. Can be used to work with RabbitMQ. -
Ratus
Ratus is a RESTful asynchronous task queue server. It translated concepts of distributed task queues into a set of resources that conform to REST principles and provides a consistent HTTP API for various backends. -
RapidMQ
RapidMQ is a pure, extremely productive, lightweight and reliable library for managing of the local messages queue
InfluxDB - Power Real-Time Data Analytics at Scale
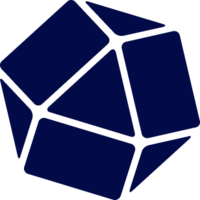
Do you think we are missing an alternative of go-events or a related project?
Popular Comparisons
README
Simple EventEmmiter for Go Programming Language. Inspired by Nodejs EventEmitter.
Overview
New() EventEmmiter // New returns a new, empty, EventEmmiter
// AddListener is an alias for .On(eventName, listener).
AddListener(EventName, ...Listener)
// Emit fires a particular event,
// Synchronously calls each of the listeners registered for the event named
// eventName, in the order they were registered,
// passing the supplied arguments to each.
Emit(EventName, ...interface{})
// EventNames returns an array listing the events for which the emitter has registered listeners.
// The values in the array will be strings.
EventNames() []EventName
// GetMaxListeners returns the max listeners for this emmiter
// see SetMaxListeners
GetMaxListeners() int
// ListenerCount returns the length of all registered listeners to a particular event
ListenerCount(EventName) int
// Listeners returns a copy of the array of listeners for the event named eventName.
Listeners(EventName) []Listener
// On registers a particular listener for an event, func receiver parameter(s) is/are optional
On(EventName, ...Listener)
// Once adds a one time listener function for the event named eventName.
// The next time eventName is triggered, this listener is removed and then invoked.
Once(EventName, ...Listener)
// RemoveAllListeners removes all listeners, or those of the specified eventName.
// Note that it will remove the event itself.
// Returns an indicator if event and listeners were found before the remove.
RemoveAllListeners(EventName) bool
// Clear removes all events and all listeners, restores Events to an empty value
Clear()
// SetMaxListeners obviously this function allows the MaxListeners
// to be decrease or increase. Set to zero for unlimited
SetMaxListeners(int)
// Len returns the length of all registered events
Len() int
import "github.com/kataras/go-events"
// initialize a new EventEmmiter to use
e := events.New()
// register an event with name "my_event" and one listener
e.On("my_event", func(payload ...interface{}) {
message := payload[0].(string)
print(message) // prints "this is my payload"
})
// fire the 'my_event' event
e.Emit("my_event", "this is my payload")
Default/global EventEmmiter
// register an event with name "my_event" and one listener to the global(package level) default EventEmmiter
events.On("my_event", func(payload ...interface{}) {
message := payload[0].(string)
print(message) // prints "this is my payload"
})
// fire the 'my_event' event
events.Emit("my_event", "this is my payload")
Remove an event
events.On("my_event", func(payload ...interface{}) {
// first listener...
},func (payload ...interface{}){
// second listener...
})
println(events.Len()) // prints 1
println(events.ListenerCount("my_event")) // prints 2
// Remove our event, when/if we don't need this or we want to clear all of its listeners
events.RemoveAllListeners("my_event")
println(events.Len()) // prints 0
println(events.ListenerCount("my_event")) // prints 0
Installation
The only requirement is the Go Programming Language.
$ go get -u github.com/kataras/go-events
FAQ
Explore these questions or navigate to the community chat.
Versioning
Current: v0.0.2
Read more about Semantic Versioning 2.0.0
- http://semver.org/
- https://en.wikipedia.org/wiki/Software_versioning
- https://wiki.debian.org/UpstreamGuide#Releases_and_Versions
People
The author of go-events is @kataras.
If you're willing to donate, feel free to send any amount through paypal
Contributing
If you are interested in contributing to the go-events project, please make a PR.
License
This project is licensed under the MIT License.
License can be found [here](LICENSE).
*Note that all licence references and agreements mentioned in the go-events README section above
are relevant to that project's source code only.