Description
Melody is websocket framework based on github.com/gorilla/websocket that abstracts away the tedious parts of handling websockets. It gets out of your way so you can write real-time apps. Features include:
melody alternatives and similar packages
Based on the "Web Frameworks" category.
Alternatively, view melody alternatives based on common mentions on social networks and blogs.
-
Gin
Gin is a HTTP web framework written in Go (Golang). It features a Martini-like API with much better performance -- up to 40 times faster. If you need smashing performance, get yourself some Gin. -
Gorilla WebSocket
DISCONTINUED. A fast, well-tested and widely used WebSocket implementation for Go. -
Iris
The fastest HTTP/2 Go Web Framework. New, modern and easy to learn. Fast development with Code you control. Unbeatable cost-performance ratio :rocket: -
GoFrame
GoFrame is a modular, powerful, high-performance and enterprise-class application development framework of Golang. -
goa
๐ Goa: Elevate Go API development! ๐ Streamlined design, automatic code generation, and seamless HTTP/gRPC support. โจ -
Faygo
Faygo is a fast and concise Go Web framework that can be used to develop high-performance web app(especially API) with fewer codes. Just define a struct handler, faygo will automatically bind/verify the request parameters and generate the online API doc. -
Huma
A modern, simple, fast & flexible micro framework for building HTTP REST/RPC APIs in Go backed by OpenAPI 3 and JSON Schema.
InfluxDB - Power Real-Time Data Analytics at Scale
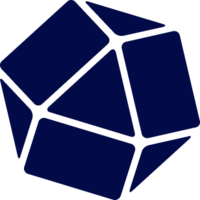
Do you think we are missing an alternative of melody or a related project?
Popular Comparisons
README
melody
:notes: Minimalist websocket framework for Go.
Melody is websocket framework based on github.com/gorilla/websocket that abstracts away the tedious parts of handling websockets. It gets out of your way so you can write real-time apps. Features include:
- [x] Clear and easy interface similar to
net/http
or Gin. - [x] A simple way to broadcast to all or selected connected sessions.
- [x] Message buffers making concurrent writing safe.
- [x] Automatic handling of sending ping/pong heartbeats that timeout broken sessions.
- [x] Store data on sessions.
Install
go get github.com/olahol/melody
Example: chat
package main
import (
"net/http"
"github.com/olahol/melody"
)
func main() {
m := melody.New()
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
http.ServeFile(w, r, "index.html")
})
http.HandleFunc("/ws", func(w http.ResponseWriter, r *http.Request) {
m.HandleRequest(w, r)
})
m.HandleMessage(func(s *melody.Session, msg []byte) {
m.Broadcast(msg)
})
http.ListenAndServe(":5000", nil)
}
Example: gophers
package main
import (
"net/http"
"strings"
"github.com/google/uuid"
"github.com/olahol/melody"
)
type GopherInfo struct {
ID, X, Y string
}
func main() {
m := melody.New()
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
http.ServeFile(w, r, "index.html")
})
http.HandleFunc("/ws", func(w http.ResponseWriter, r *http.Request) {
m.HandleRequest(w, r)
})
m.HandleConnect(func(s *melody.Session) {
ss, _ := m.Sessions()
for _, o := range ss {
value, exists := o.Get("info")
if !exists {
continue
}
info := value.(*GopherInfo)
s.Write([]byte("set " + info.ID + " " + info.X + " " + info.Y))
}
id := uuid.NewString()
s.Set("info", &GopherInfo{id, "0", "0"})
s.Write([]byte("iam " + id))
})
m.HandleDisconnect(func(s *melody.Session) {
value, exists := s.Get("info")
if !exists {
return
}
info := value.(*GopherInfo)
m.BroadcastOthers([]byte("dis "+info.ID), s)
})
m.HandleMessage(func(s *melody.Session, msg []byte) {
p := strings.Split(string(msg), " ")
value, exists := s.Get("info")
if len(p) != 2 || !exists {
return
}
info := value.(*GopherInfo)
info.X = p[0]
info.Y = p[1]
m.BroadcastOthers([]byte("set "+info.ID+" "+info.X+" "+info.Y), s)
})
http.ListenAndServe(":5000", nil)
}
More examples
Documentation
Contributors
FAQ
If you are getting a 403
when trying to connect to your websocket you can change allow all origin hosts:
m := melody.New()
m.Upgrader.CheckOrigin = func(r *http.Request) bool { return true }