jazz alternatives and similar packages
Based on the "Messaging" category.
Alternatively, view jazz alternatives based on common mentions on social networks and blogs.
-
sarama
DISCONTINUED. Sarama is a Go library for Apache Kafka. [Moved to: https://github.com/IBM/sarama] -
Centrifugo
Scalable real-time messaging server in a language-agnostic way. Self-hosted alternative to Pubnub, Pusher, Ably. Set up once and forever. -
APNs2
⚡ HTTP/2 Apple Push Notification Service (APNs) push provider for Go — Send push notifications to iOS, tvOS, Safari and OSX apps, using the APNs HTTP/2 protocol. -
Uniqush-Push
Uniqush is a free and open source software system which provides a unified push service for server side notification to apps on mobile devices. -
amqp
An AMQP 0-9-1 Go client maintained by the RabbitMQ team. Originally by @streadway: `streadway/amqp` -
Chanify
Chanify is a safe and simple notification tools. This repository is command line tools for Chanify. -
PingMe
PingMe is a CLI which provides the ability to send messages or alerts to multiple messaging platforms & email. -
emitter
Emits events in Go way, with wildcard, predicates, cancellation possibilities and many other good wins -
Bus
🔊Minimalist message bus implementation for internal communication with zero-allocation magic on Emit -
go-mq
Declare AMQP entities like queues, producers, and consumers in a declarative way. Can be used to work with RabbitMQ. -
Ratus
Ratus is a RESTful asynchronous task queue server. It translated concepts of distributed task queues into a set of resources that conform to REST principles and provides a consistent HTTP API for various backends. -
RapidMQ
RapidMQ is a pure, extremely productive, lightweight and reliable library for managing of the local messages queue
WorkOS - The modern identity platform for B2B SaaS
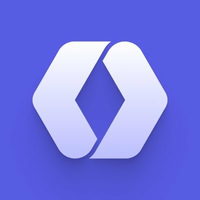
Do you think we are missing an alternative of jazz or a related project?
README
Jazz
Abstraction layer for quick and simple rabbitMQ connection, messaging and administration. Inspired by Jazz Jackrabbit and his eternal hatred towards slow turtles.
Usage
This library contains three major parts - exchange/queue scheme creation, publishing of messages and consuming of messages. The greatest benefit of this partitioning is that each part might be in separate application. Also due to dedicated administration part, publishing and consuming of messages is simplified to great extent.
Step 1: Connect to rabbit
import(
"github.com/socifi/jazz"
)
var dsn = "amqp://guest:guest@localhost:5672/"
func main() {
// ...
c, err := jazz.Connect(dsn)
if err != nil {
t.Errorf("Could not connect to RabbitMQ: %v", err.Error())
return
}
//...
}
Step 2: Create scheme
Scheme specification is done via structure Settings
which can be easily specified in YAML. So generally you need to decode YAML and then create all queues and exchanges
It can be something really crazy like this!
var data = []byte(`
exchanges:
exchange0:
durable: true
type: topic
exchange1:
durable: true
type: topic
bindings:
- exchange: "exchange0"
key: "key1"
- exchange: "exchange0"
key: "key2"
exchange2:
durable: true
type: topic
bindings:
- exchange: "exchange0"
key: "key3"
- exchange: "exchange1"
key: "key2"
exchange3:
durable: true
type: topic
bindings:
- exchange: "exchange0"
key: "key4"
queues:
queue0:
durable: true
bindings:
- exchange: "exchange0"
key: "key4"
queue1:
durable: true
bindings:
- exchange: "exchange1"
key: "key2"
queue2:
durable: true
bindings:
- exchange: "exchange1"
key: "#"
queue3:
durable: true
bindings:
- exchange: "exchange2"
key: "#"
queue4:
durable: true
bindings:
- exchange: "exchange3"
key: "#"
queue5:
durable: true
bindings:
- exchange: "exchange0"
key: "#"
`)
func main() {
// ...
reader := bytes.NewReader(data)
scheme, err := DecodeYaml(reader)
if err != nil {
t.Errorf("Could not read YAML: %v", err.Error())
return
}
err = c.CreateScheme(scheme)
if err != nil {
t.Errorf("Could not create scheme: %v", err.Error())
return
}
//...
// Be nice and delete scheme (Not advisable in ).
err = c.DeleteScheme(scheme)
if err != nil {
t.Errorf("Could not delete scheme: %v", err.Error())
return
}
}
Step 3: Publish and/or consume messages
You can process each queue in separate application or everything together like this:
func main() {
// ...
f := func(msg []byte) {
fmt.Println(string(msg))
}
go c.ProcessQueue("queue1", f)
go c.ProcessQueue("queue2", f)
go c.ProcessQueue("queue3", f)
go c.ProcessQueue("queue4", f)
go c.ProcessQueue("queue5", f)
go c.ProcessQueue("queue6", f)
c.SendMessage("exchange0", "key1", "Hello World!")
c.SendMessage("exchange0", "key2", "Hello!")
c.SendMessage("exchange0", "key3", "World!")
c.SendMessage("exchange0", "key4", "Hi!")
c.SendMessage("exchange0", "key5", "Again!")
//...
}
Notes
No copyright infringement intended. The name Jazz Jackrabbit and artwork of Jazz Jackrabbit is intelectual property of Epic MegaGames and was taken over from wikipedia