go-lark alternatives and similar packages
Based on the "Third-party APIs" category.
Alternatively, view lark alternatives based on common mentions on social networks and blogs.
-
githubql
Package githubv4 is a client library for accessing GitHub GraphQL API v4 (https://docs.github.com/en/graphql). -
openaigo
OpenAI GPT3/3.5 and GPT4 ChatGPT API Client Library for Go, simple, less dependencies, and well-tested -
gostorm
GoStorm is a Go library that implements the communications protocol required to write Storm spouts and Bolts in Go that communicate with the Storm shells. -
ynab
Go client for the YNAB API. Unofficial. It covers 100% of the resources made available by the YNAB API.
InfluxDB - Power Real-Time Data Analytics at Scale
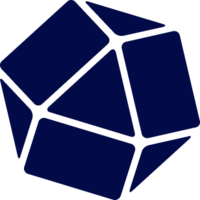
Do you think we are missing an alternative of go-lark or a related project?
README
go-lark
go-lark is an easy-to-use SDK for Feishu and Lark Open Platform, which implements messaging APIs, with full-fledged supports on building Chat Bot and Notification Bot.
It is widely used and tested by ~450 ByteDance in-house developers with over 1.5k Go packages.
Features
- Notification bot & chat bot supported
- Send messages (Group, Private, Rich Text, and Card)
- Quick to build message with
MsgBuffer
- Easy to create incoming message hook
- Encryption and token verification supported
- Middleware support for Gin web framework
- Highly extensible
- Documentation & tests
Installation
go get github.com/go-lark/lark
Quick Start
Prerequisite
There are two types of bot that is supported by go-lark. We need to create a bot manually.
Chat Bot:
- Feishu: create from Feishu Open Platform.
- Lark: create from Lark Developer.
- App ID and App Secret are required to init a
ChatBot
.
Notification Bot:
- Create from group chat.
- Web Hook URL is required.
Sending Message
Chat Bot:
import "github.com/go-lark/lark"
func main() {
bot := lark.NewChatBot("<App ID>", "<App Secret>")
bot.StartHeartbeat()
bot.PostText("hello, world", lark.WithEmail("[email protected]"))
}
Notification Bot:
import "github.com/go-lark/lark"
func main() {
bot := lark.NewNotificationBot("<WEB HOOK URL>")
bot.PostNotification("title", "text")
}
Feishu/Lark API offers more features, please refers to Usage for further documentation.
Limits
- go-lark is tested on Feishu endpoints, which literally compats Lark endpoints, because Feishu and Lark basically shares the same API specification. We do not guarantee all of the APIs work well with Lark, until we have tested it on Lark.
- go-lark only supports Custom App. Marketplace App is not supported yet.
- go-lark implements bot and messaging API, other APIs such as Lark Doc, Calendar and so so are not supported.
- go-lark implements API in v3/v4 version* (official documents may also mention im/v1 version) and event with Schema 1.0.
Switch to Lark Endpoints
The default API endpoints are for Feishu, in order to switch to Lark, we should use SetDomain
:
bot := lark.NewChatBot("<App ID>", "<App Secret>")
bot.SetDomain(lark.DomainLark)
Usage
Auth
Auto-renewable authentication:
// initialize a chat bot with appID and appSecret
bot := lark.NewChatBot(appID, appSecret)
// Renew access token periodically
bot.StartHeartbeat()
// Stop renewal
bot.StopHeartbeat()
Single-pass authentication:
bot := lark.NewChatBot(appID, appSecret)
resp, err := bot.GetTenantAccessTokenInternal(true)
// and we can now access the token value with `bot.tenantAccessToken()`
Example: examples/auth
Messaging
For Chat Bot, we can send simple messages with the following method:
PostText
PostTextMention
PostTextMentionAll
PostImage
PostShareChatCard
Basic message examples: examples/basic-message
To build rich messages, we may use Message Buffer (or simply MsgBuffer
),
which builds message conveniently with chaining methods.
Examples
Apart from the general auth and messaging chapter, there are comprehensive examples for almost all APIs.
Here is a collection of ready-to-run examples for each part of go-lark
:
- examples/auth
- examples/basic-message
- examples/image-message
- examples/rich-text-message
- examples/share-chat
- examples/interactive-message
- examples/group
Message Buffer
We can build message body with MsgBuffer
and send with PostMessage
, which supports the following message types:
MsgText
: TextMsgPost
: Rich TextMsgInteractive
: Interactive CardMsgShareCard
: Group Share CardMsgShareUser
: User Share CardMsgImage
: ImageMsgFile
: FileMsgAudio
: AudioMsgMedia
: MediaMsgSticker
: Sticker
MsgBuffer
provides binding functions and content functions.
Binding functions:
Function | Usage | Comment |
---|---|---|
BindChatID | Bind a chat ID | Either OpenID , UserID , Email , ChatID or UnionID should be present |
BindOpenID | Bind a user open ID | |
BindUserID | Bind a user ID | |
BindUnionID | Bind a union ID | |
BindEmail | Bind a user email | |
BindReply | Bind a reply ID | Required when reply a message |
Content functions pair with message content types. If it mismatched, it would not have sent successfully. Content functions:
Function | Message Type | Usage | Comment |
---|---|---|---|
Text | MsgText |
Append plain text | May build with TextBuilder |
Post | MsgPost |
Append rich text | May build with PostBuilder |
Card | MsgInteractive |
Append interactive card | May build with [CardBuilder ](card/README.md) |
ShareChat | MsgShareCard |
Append group share card | |
ShareUser | MsgShareUser |
Append user share card | |
Image | MsgImage |
Append image | Required to upload to Lark server in advance |
File | MsgFile |
Append file | Required to upload to Lark server in advance |
Audio | MsgAudio |
Append audio | Required to upload to Lark server in advance |
Media | MsgMedia |
Append media | Required to upload to Lark server in advance |
Sticker | MsgSticker |
Append sticker | Required to upload to Lark server in advance |
Error Handling
Each go-lark
API function returns response
and err
.
err
is the error from HTTP client, when it was not nil
, HTTP might have gone wrong.
While response
is HTTP response from Lark API server, in which Code
and Ok
represent whether it succeeds.
The meaning of Code
is defined here.
Event
Lark provides a number of events and they are in two different schema (1.0/2.0). go-lark now only implements two of them in schema 1.0, which are needed for interacting between bot and Lark server:
- URL Challenge
- Receiving Messages
We recommend Gin middleware to handle these events.
Gin Middleware
Example: examples/gin-middleware
URL Challenge
r := gin.Default()
middleware := larkgin.NewLarkMiddleware()
middleware.BindURLPrefix("/handle") // supposed URL is http://your.domain.com/handle
r.Use(middleware.LarkChallengeHandler())
Event V2
Lark has provided event v2 and it applied automatically to newly created bots.
r := gin.Default()
middleware := larkgin.NewLarkMiddleware()
r.Use(middleware.LarkEventHandler())
Get the event (e.g. Message):
r.POST("/", func(c *gin.Context) {
if evt, ok := middleware.GetEvent(c); ok { // => GetEvent instead of GetMessage
if evt.Header.EventType == lark.EventTypeMessageReceived {
if msg, err := evt.GetMessageReceived(); err == nil {
fmt.Println(msg.Message.Content)
}
}
}
})
Receiving Message
For older bots, please use v1:
r := gin.Default()
middleware := larkgin.NewLarkMiddleware()
middleware.BindURLPrefix("/handle") // supposed URL is http://your.domain.com/handle
r.POST("/handle", func(c *gin.Context) {
if msg, ok := middleware.GetMessage(c); ok && msg != nil {
text := msg.Event.Text
// your awesome logic
}
})
Security & Encryption
Lark Open Platform offers AES encryption and token verification to ensure security for events.
- AES Encryption: when switch on, all traffic will be encrypted with AES.
- Token Verification: simple token verification for incoming messages.
We recommend you to enable token verification. If HTTPS is not available on your host, then enable AES encryption.
middleware.WithTokenVerfication("<verification-token>")
middleware.WithEncryption("<encryption-key>")
Debugging
Lark does not provide messaging API debugger officially. Thus, we have to debug with real Lark conversation.
We add PostEvent
to simulate message sending to make it easier.
PostEvent
can also be used to redirect message, which acts like a reverse proxy.
Example: examples/event-forward
Notice:
PostEvent
does not support AES encryption at the moment.
Development
Test
- Dotenv Setup
go-lark uses godotenv
test locally. You may have to create a .env
file in repo directory, which contains environmental variables:
LARK_APP_ID
LARK_APP_SECRET
LARK_USER_EMAIL
LARK_USER_ID
LARK_UNION_ID
LARK_OPEN_ID
LARK_CHAT_ID
LARK_MESSAGE_ID
LARK_APP_ID
and LARK_APP_SECRET
are mandatory. Others are required only by specific API tests.
- Run Test
GO_LARK_TEST_MODE=local ./scripts/test.sh
Extensions
go-lark's dev utilities (authentication, HTTP handling, and etc.) are capable for easily implementing most of APIs provided by Lark Open Platform. And we may use that as an extension for go-lark.
Here is an example that implementing a Lark Doc API with go-lark:
package lark
import "github.com/go-lark/lark"
const copyFileAPIPattern = "/open-apis/drive/explorer/v2/file/copy/files/%s"
// CopyFileResponse .
type CopyFileResponse struct {
lark.BaseResponse
Data CopyFileData `json:"data"`
}
// CopyFileData .
type CopyFileData struct {
FolderToken string `json:"folderToken"`
Revision int64 `json:"revision"`
Token string `json:"token"`
Type string `json:"type"`
URL string `json:"url"`
}
// CopyFile implementation
func CopyFile(bot *lark.Bot, fileToken, dstFolderToken, dstName string) (*CopyFileResponse, error) {
var respData model.CopyFileResponse
err := bot.PostAPIRequest(
"CopyFile",
fmt.Sprintf(copyFileAPIPattern, fileToken),
true,
map[string]interface{}{
"type": "doc",
"dstFolderToken": dstFolderToken,
"dstName": dstName,
"permissionNeeded": true,
"CommentNeeded": false,
},
&respData,
)
return &respData, err
}
FAQ
- I got
99991401
when sending messages- remove IP Whitelist from dashboard
- My bot failed sending messages
- check authentication.
- not invite to the group.
- API permission not applied.
- Does go-lark support interactive message card?
- Yes, use card builder.
Contributing
- If you think you've found a bug with go-lark, please file an issue.
- Pull Request is welcomed.
License
Copyright (c) David Zhang, 2018-2022. Licensed under MIT License.
*Note that all licence references and agreements mentioned in the go-lark README section above
are relevant to that project's source code only.