douceur alternatives and similar packages
Based on the "Email" category.
Alternatively, view douceur alternatives based on common mentions on social networks and blogs.
-
go-simple-mail
Golang package for send email. Support keep alive connection, TLS and SSL. Easy for bulk SMTP. -
Mailchain
Using Mailchain, blockchain users can now send and receive rich-media HTML messages with attachments via a blockchain address. -
truemail-go
๐ Configurable Golang ๐จ email validator/verifier. Verify email via Regex, DNS, SMTP and even more. Be sure that email address valid and exists. -
go-mail
:incoming_envelope: Simple email interface across multiple service providers (ses, postmark, mandrill, smtp)
InfluxDB - Power Real-Time Data Analytics at Scale
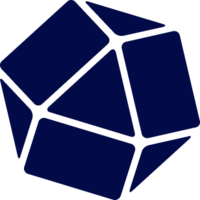
Do you think we are missing an alternative of douceur or a related project?
Popular Comparisons
README
douceur 
A simple CSS parser and inliner in Golang.
Parser is vaguely inspired by CSS Syntax Module Level 3 and corresponding JS parser.
Inliner only parses CSS defined in HTML document, it DOES NOT fetch external stylesheets (for now).
Inliner inserts additional attributes when possible, for example:
<html>
<head>
<style type="text/css">
body {
background-color: #f2f2f2;
}
</style>
</head>
<body>
<p>Inline me !</p>
</body>
</html>`
Becomes:
<html>
<head>
</head>
<body style="background-color: #f2f2f2;" bgcolor="#f2f2f2">
<p>Inline me !</p>
</body>
</html>`
The bgcolor
attribute is inserted, in addition to the inlined background-color
style.
Tool usage
Install tool:
$ go install github.com/aymerick/douceur
Parse a CSS file and display result:
$ douceur parse inputfile.css
Inline CSS in an HTML document and display result:
$ douceur inline inputfile.html
Library usage
Fetch package:
$ go get github.com/aymerick/douceur
Parse CSS
package main
import (
"fmt"
"github.com/aymerick/douceur/parser"
)
func main() {
input := `body {
/* D4rK s1T3 */
background-color: black;
}
p {
/* Try to read that ! HAHA! */
color: red; /* L O L */
}
`
stylesheet, err := parser.Parse(input)
if err != nil {
panic("Please fill a bug :)")
}
fmt.Print(stylesheet.String())
}
Displays:
body {
background-color: black;
}
p {
color: red;
}
Inline HTML
package main
import (
"fmt"
"github.com/aymerick/douceur/inliner"
)
func main() {
input := `<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<style type="text/css">
p {
font-family: 'Helvetica Neue', Verdana, sans-serif;
color: #eee;
}
</style>
</head>
<body>
<p>
Inline me please!
</p>
</body>
</html>`
html, err := inliner.Inline(input)
if err != nil {
panic("Please fill a bug :)")
}
fmt.Print(html)
}
Displays:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"><html xmlns="http://www.w3.org/1999/xhtml"><head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
</head>
<body>
<p style="color: #eee; font-family: 'Helvetica Neue', Verdana, sans-serif;">
Inline me please!
</p>
</body></html>
Test
go test ./... -v
Dependencies
- Parser uses Gorilla CSS3 tokenizer.
- Inliner uses [goquery](github.com/PuerkitoBio/goquery) to manipulate HTML.