sqlingo alternatives and similar packages
Based on the "Database" category.
Alternatively, view sqlingo alternatives based on common mentions on social networks and blogs.
-
tidb
TiDB is an open-source, cloud-native, distributed, MySQL-Compatible database for elastic scale and real-time analytics. Try AI-powered Chat2Query free at : https://tidbcloud.com/free-trial -
TinyGo
Go compiler for small places. Microcontrollers, WebAssembly (WASM/WASI), and command-line tools. Based on LLVM. -
groupcache
groupcache is a caching and cache-filling library, intended as a replacement for memcached in many cases. -
bytebase
The GitLab/GitHub for database DevOps. World's most advanced database DevOps and CI/CD for Developer, DBA and Platform Engineering teams. -
go-cache
An in-memory key:value store/cache (similar to Memcached) library for Go, suitable for single-machine applications. -
immudb
immudb - immutable database based on zero trust, SQL/Key-Value/Document model, tamperproof, data change history -
buntdb
BuntDB is an embeddable, in-memory key/value database for Go with custom indexing and geospatial support -
pREST
PostgreSQL ➕ REST, low-code, simplify and accelerate development, ⚡ instant, realtime, high-performance on any Postgres application, existing or new -
xo
Command line tool to generate idiomatic Go code for SQL databases supporting PostgreSQL, MySQL, SQLite, Oracle, and Microsoft SQL Server -
nutsdb
A simple, fast, embeddable, persistent key/value store written in pure Go. It supports fully serializable transactions and many data structures such as list, set, sorted set. -
gocraft/dbr (database records)
Additions to Go's database/sql for super fast performance and convenience. -
lotusdb
Most advanced key-value database written in Go, extremely fast, compatible with LSM tree and B+ tree.
WorkOS - The modern identity platform for B2B SaaS
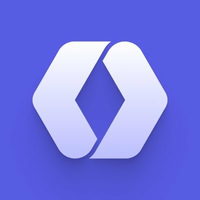
Do you think we are missing an alternative of sqlingo or a related project?
Popular Comparisons
README
sqlingo is a SQL DSL (a.k.a. SQL Builder or ORM) library in Go. It generates code from the database and lets you write SQL queries in an elegant way.
Features
- Auto-generating DSL objects and model structs from the database so you don't need to manually keep things in sync
- SQL DML (SELECT / INSERT / UPDATE / DELETE) with some advanced SQL query syntaxes
- Many common errors could be detected at compile time
- Your can use the features in your editor / IDE, such as autocompleting the fields and queries, or finding the usage of a field or a table
- Context support
- Transaction support
- Interceptor support
Database Support Status
Database | Status |
---|---|
MySQL | stable |
PostgreSQL | experimental |
SQLite | experimental |
Tutorial
Install and use sqlingo code generator
The first step is to generate code from the database. In order to generate code, sqlingo requires your tables are already created in the database.
$ go get -u github.com/lqs/sqlingo/sqlingo-gen-mysql
$ mkdir -p generated/sqlingo
$ sqlingo-gen-mysql root:123456@/database_name >generated/sqlingo/database_name.dsl.go
Write your application
Here's a demonstration of some simple & advanced usage of sqlingo.
package main
import (
"github.com/lqs/sqlingo"
. "./generated/sqlingo"
)
func main() {
db, err := sqlingo.Open("mysql", "root:123456@/database_name")
if err != nil {
panic(err)
}
// a simple query
var customers []*CustomerModel
db.SelectFrom(Customer).
Where(Customer.Id.In(1, 2)).
OrderBy(Customer.Name.Desc()).
FetchAll(&customers)
// query from multiple tables
var customerId int64
var orderId int64
err = db.Select(Customer.Id, Order.Id).
From(Customer, Order).
Where(Customer.Id.Equals(Order.CustomerId), Order.Id.Equals(1)).
FetchFirst(&customerId, &orderId)
// subquery and count
count, err := db.SelectFrom(Order)
Where(Order.CustomerId.In(db.Select(Customer.Id).
From(Customer).
Where(Customer.Name.Equals("Customer One")))).
Count()
// group-by with auto conversion to map
var customerIdToOrderCount map[int64]int64
err = db.Select(Order.CustomerId, f.Count(1)).
From(Order).
GroupBy(Order.CustomerId).
FetchAll(&customerIdToOrderCount)
if err != nil {
println(err)
}
// insert some rows
customer1 := &CustomerModel{name: "Customer One"}
customer2 := &CustomerModel{name: "Customer Two"}
_, err = db.InsertInto(Customer).
Models(customer1, customer2).
Execute()
// insert with on-duplicate-key-update
_, err = db.InsertInto(Customer).
Fields(Customer.Id, Customer.Name).
Values(42, "Universe").
OnDuplicateKeyUpdate().
Set(Customer.Name, Customer.Name.Concat(" 2")).
Execute()
}
*Note that all licence references and agreements mentioned in the sqlingo README section above
are relevant to that project's source code only.