go-jira alternatives and similar packages
Based on the "Third-party APIs" category.
Alternatively, view go-jira alternatives based on common mentions on social networks and blogs.
-
githubql
Package githubv4 is a client library for accessing GitHub GraphQL API v4 (https://docs.github.com/en/graphql). -
openaigo
OpenAI GPT3/3.5 and GPT4 ChatGPT API Client Library for Go, simple, less dependencies, and well-tested -
gostorm
GoStorm is a Go library that implements the communications protocol required to write Storm spouts and Bolts in Go that communicate with the Storm shells. -
ynab
Go client for the YNAB API. Unofficial. It covers 100% of the resources made available by the YNAB API.
WorkOS - The modern identity platform for B2B SaaS
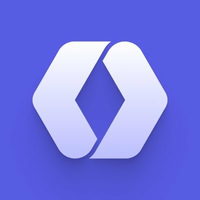
Do you think we are missing an alternative of go-jira or a related project?
README
go-jira
Go client library for Atlassian Jira.
[Go client library for Atlassian Jira](./img/logo_small.png "Go client library for Atlassian Jira.")
:warning: State of this library :warning:
v2 of this library is in development. v2 will contain breaking changes :warning: The current main branch can contains the development version of v2.
The goals of v2 are:
- idiomatic go usage
- proper documentation
- being compliant with different kinds of Atlassian Jira products (on-premise vs. cloud)
- remove flaws introduced during the early times of this library
See our milestone Road to v2 and provide feedback in Development is kicking: Road to v2 🚀 #489.
Attention: The current main
branch represents the v2 development version - we treat this version as unstable and breaking changes are expected.
If you want to stay more stable, please use v1.* - See our releases. Latest stable release: v1.16.0
Features
- Authentication (HTTP Basic, OAuth, Session Cookie)
- Create and retrieve issues
- Create and retrieve issue transitions (status updates)
- Call every API endpoint of the Jira, even if it is not directly implemented in this library
This package is not Jira API complete (yet), but you can call every API endpoint you want. See Call a not implemented API endpoint how to do this. For all possible API endpoints of Jira have a look at latest Jira REST API documentation.
Requirements
- Go >= 1.14
- Jira v6.3.4 & v7.1.2.
Note that we also run our tests against 1.13, though only the last two versions of Go are officially supported.
Installation
It is go gettable
go get github.com/andygrunwald/go-jira
For stable versions you can use one of our tags with gopkg.in. E.g.
package main
import (
jira "gopkg.in/andygrunwald/go-jira.v1"
)
...
(optional) to run unit / example tests:
cd $GOPATH/src/github.com/andygrunwald/go-jira
go test -v ./...
API
Please have a look at the GoDoc documentation for a detailed API description.
The latest Jira REST API documentation was the base document for this package.
Examples
Further a few examples how the API can be used. A few more examples are available in the GoDoc examples section.
Get a single issue
Lets retrieve MESOS-3325 from the Apache Mesos project.
package main
import (
"fmt"
jira "github.com/andygrunwald/go-jira"
)
func main() {
jiraClient, _ := jira.NewClient(nil, "https://issues.apache.org/jira/")
issue, _, _ := jiraClient.Issue.Get("MESOS-3325", nil)
fmt.Printf("%s: %+v\n", issue.Key, issue.Fields.Summary)
fmt.Printf("Type: %s\n", issue.Fields.Type.Name)
fmt.Printf("Priority: %s\n", issue.Fields.Priority.Name)
// MESOS-3325: Running [email protected] in a container causes slave to be lost after a restart
// Type: Bug
// Priority: Critical
}
Authentication
The go-jira
library does not handle most authentication directly. Instead, authentication should be handled within
an http.Client
. That client can then be passed into the NewClient
function when creating a jira client.
For convenience, capability for basic and cookie-based authentication is included in the main library.
Token (Jira on Atlassian Cloud)
Token-based authentication uses the basic authentication scheme, with a user-generated API token in place of a user's password. You can generate a token for your user here. Additional information about Atlassian Cloud API tokens can be found here.
A more thorough, [runnable example](examples/basicauth/main.go) is provided in the examples directory.
func main() {
tp := jira.BasicAuthTransport{
Username: "username",
Password: "token",
}
client, err := jira.NewClient(tp.Client(), "https://my.jira.com")
u, _, err := client.User.Get("some_user")
fmt.Printf("\nEmail: %v\nSuccess!\n", u.EmailAddress)
}
Basic (self-hosted Jira)
Password-based API authentication works for self-hosted Jira only, and has been deprecated for users of Atlassian Cloud.
The above token authentication example may be used, substituting a user's password for a generated token.
Authenticate with OAuth
If you want to connect via OAuth to your Jira Cloud instance checkout the example of using OAuth authentication with Jira in Go by @Lupus.
For more details have a look at the issue #56.
Create an issue
Example how to create an issue.
package main
import (
"fmt"
"github.com/andygrunwald/go-jira"
)
func main() {
base := "https://my.jira.com"
tp := jira.BasicAuthTransport{
Username: "username",
Password: "token",
}
jiraClient, err := jira.NewClient(tp.Client(), base)
if err != nil {
panic(err)
}
i := jira.Issue{
Fields: &jira.IssueFields{
Assignee: &jira.User{
Name: "myuser",
},
Reporter: &jira.User{
Name: "youruser",
},
Description: "Test Issue",
Type: jira.IssueType{
Name: "Bug",
},
Project: jira.Project{
Key: "PROJ1",
},
Summary: "Just a demo issue",
},
}
issue, _, err := jiraClient.Issue.Create(&i)
if err != nil {
panic(err)
}
fmt.Printf("%s: %+v\n", issue.Key, issue.Fields.Summary)
}
Change an issue status
This is how one can change an issue status. In this example, we change the issue from "To Do" to "In Progress."
package main
import (
"fmt"
"github.com/andygrunwald/go-jira"
)
func main() {
base := "https://my.jira.com"
tp := jira.BasicAuthTransport{
Username: "username",
Password: "token",
}
jiraClient, err := jira.NewClient(tp.Client(), base)
if err != nil {
panic(err)
}
issue, _, _ := jiraClient.Issue.Get("FART-1", nil)
currentStatus := issue.Fields.Status.Name
fmt.Printf("Current status: %s\n", currentStatus)
var transitionID string
possibleTransitions, _, _ := jiraClient.Issue.GetTransitions("FART-1")
for _, v := range possibleTransitions {
if v.Name == "In Progress" {
transitionID = v.ID
break
}
}
jiraClient.Issue.DoTransition("FART-1", transitionID)
issue, _, _ = jiraClient.Issue.Get(testIssueID, nil)
fmt.Printf("Status after transition: %+v\n", issue.Fields.Status.Name)
}
Get all the issues for JQL with Pagination
Jira API has limit on maxResults it can return. You may have a usecase where you need to get all issues for given JQL. This example shows reference implementation of GetAllIssues function which does pagination on Jira API to get all the issues for given JQL
please look at Pagination Example
Call a not implemented API endpoint
Not all API endpoints of the Jira API are implemented into go-jira. But you can call them anyway: Lets get all public projects of Atlassian`s Jira instance.
package main
import (
"fmt"
"github.com/andygrunwald/go-jira"
)
func main() {
base := "https://my.jira.com"
tp := jira.BasicAuthTransport{
Username: "username",
Password: "token",
}
jiraClient, err := jira.NewClient(tp.Client(), base)
req, _ := jiraClient.NewRequest("GET", "rest/api/2/project", nil)
projects := new([]jira.Project)
_, err = jiraClient.Do(req, projects)
if err != nil {
panic(err)
}
for _, project := range *projects {
fmt.Printf("%s: %s\n", project.Key, project.Name)
}
// ...
// BAM: Bamboo
// BAMJ: Bamboo Jira Plugin
// CLOV: Clover
// CONF: Confluence
// ...
}
Implementations
- andygrunwald/jitic - The Jira Ticket Checker
Code structure
The code structure of this package was inspired by google/go-github.
There is one main part (the client).
Based on this main client the other endpoints, like Issues or Authentication are extracted in services. E.g. IssueService
or AuthenticationService
.
These services own a responsibility of the single endpoints / usecases of Jira.
Contribution
We ❤️ PR's
Contribution, in any kind of way, is highly welcome! It doesn't matter if you are not able to write code. Creating issues or holding talks and help other people to use go-jira is contribution, too! A few examples:
- Correct typos in the README / documentation
- Reporting bugs
- Implement a new feature or endpoint
- Sharing the love of go-jira and help people to get use to it
If you are new to pull requests, checkout Collaborating on projects using issues and pull requests / Creating a pull request.
Supported Go versions
We follow the Go Release Policy:
Each major Go release is supported until there are two newer major releases. For example, Go 1.5 was supported until the Go 1.7 release, and Go 1.6 was supported until the Go 1.8 release. We fix critical problems, including critical security problems, in supported releases as needed by issuing minor revisions (for example, Go 1.6.1, Go 1.6.2, and so on).
Supported Jira versions
Jira Server (On-Premise solution)
We follow the Atlassian Support End of Life Policy:
Atlassian supports feature versions for two years after the first major iteration of that version was released (for example, we support Jira Core 7.2.x for 2 years after Jira 7.2.0 was released).
Jira Cloud
- Jira Cloud API in version 2
Jira Cloud API in version 3 is currently not officially supported, because it is still in beta.
Official Jira API documentation
- Jira Server (On-Premise solution)
- Jira Cloud API in version 2
- Jira Cloud API in version 3
Sandbox environment for testing
Jira offers sandbox test environments at http://go.atlassian.com/cloud-dev.
You can read more about them at https://developer.atlassian.com/blog/2016/04/cloud-ecosystem-dev-env/.
Releasing
Install standard-version
npm i -g standard-version
standard-version
git push --tags
Manually copy/paste text from changelog (for this new version) into the release on Github.com. E.g.
https://github.com/andygrunwald/go-jira/releases/edit/v1.11.0
License
This project is released under the terms of the MIT license.
*Note that all licence references and agreements mentioned in the go-jira README section above
are relevant to that project's source code only.