Popularity
4.3
Declining
Activity
0.0
Stable
83
7
24
Programming language: Go
Tags:
Data Structures
skiplist alternatives and similar packages
Based on the "Data Structures" category.
Alternatively, view skiplist alternatives based on common mentions on social networks and blogs.
-
golang-set
A simple, battle-tested and generic set type for the Go language. Trusted by Docker, 1Password, Ethereum and Hashicorp. -
hyperloglog
HyperLogLog with lots of sugar (Sparse, LogLog-Beta bias correction and TailCut space reduction) brought to you by Axiom -
ttlcache
DISCONTINUED. An in-memory cache with item expiration and generics [Moved to: https://github.com/jellydator/ttlcache] -
Bloomfilter
DISCONTINUED. Face-meltingly fast, thread-safe, marshalable, unionable, probability- and optimal-size-calculating Bloom filter in go -
hilbert
DISCONTINUED. Go package for mapping values to and from space-filling curves, such as Hilbert and Peano curves. -
cuckoo-filter
Cuckoo Filter go implement, better than Bloom Filter, configurable and space optimized 布谷鸟过滤器的Go实现,优于布隆过滤器,可以定制化过滤器参数,并进行了空间优化 -
go-rquad
:pushpin: State of the art point location and neighbour finding algorithms for region quadtrees, in Go -
nan
Zero allocation Nullable structures in one library with handy conversion functions, marshallers and unmarshallers
WorkOS - The modern identity platform for B2B SaaS
The APIs are flexible and easy-to-use, supporting authentication, user identity, and complex enterprise features like SSO and SCIM provisioning.
Promo
workos.com
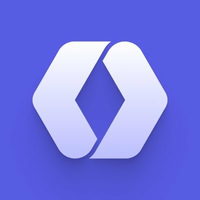
Do you think we are missing an alternative of skiplist or a related project?
README
skiplist
reference from redis zskiplist
Usage
package main
import (
"fmt"
"github.com/gansidui/skiplist"
"log"
)
type User struct {
score float64
id string
}
func (u *User) Less(other interface{}) bool {
if u.score > other.(*User).score {
return true
}
if u.score == other.(*User).score && len(u.id) > len(other.(*User).id) {
return true
}
return false
}
func main() {
us := make([]*User, 7)
us[0] = &User{6.6, "hi"}
us[1] = &User{4.4, "hello"}
us[2] = &User{2.2, "world"}
us[3] = &User{3.3, "go"}
us[4] = &User{1.1, "skip"}
us[5] = &User{2.2, "list"}
us[6] = &User{3.3, "lang"}
// insert
sl := skiplist.New()
for i := 0; i < len(us); i++ {
sl.Insert(us[i])
}
// traverse
for e := sl.Front(); e != nil; e = e.Next() {
fmt.Println(e.Value.(*User).id, "-->", e.Value.(*User).score)
}
fmt.Println()
// rank
rank1 := sl.GetRank(&User{2.2, "list"})
rank2 := sl.GetRank(&User{6.6, "hi"})
if rank1 != 6 || rank2 != 1 {
log.Fatal()
}
if e := sl.GetElementByRank(2); e.Value.(*User).score != 4.4 || e.Value.(*User).id != "hello" {
log.Fatal()
}
}
/* output:
hi --> 6.6
hello --> 4.4
lang --> 3.3
go --> 3.3
world --> 2.2
list --> 2.2
skip --> 1.1
*/
License
MIT
*Note that all licence references and agreements mentioned in the skiplist README section above
are relevant to that project's source code only.